Aim:
Develop Programming Working With Font.
Procedure:
- Step 1: Open a New Java Application on Netbeans Editor.
- Step 2: Open Netbeans Ide, Choose File new Project.
- Step 3: New Open a New Project and Choose a Java Application.
- Step 4: Add a Coding on Coding Windows Using Getter Setter Method.
- Step 5: Run the Index Page and Verify the Result Page.
- Step 6: Save the Project (Ctrl+s).
- Step 7: Run the Project (F5).
Program:
package ex3; import java.awt.*; public class Display extends Frame { static String[] fonts; private Dimension dim; Display () { super ("Font Display"); fonts = Toolkit.getDefaultToolkit().getFontList(); } public void addNotify() { Font f; super.addNotify(); int height = 0; int maxWidth = 0; final int vMargin = 5, hMargin = 5; for (int i=0;i<fonts.length;i++) { f = new Font (fonts[i], Font.PLAIN, 12); height += getHeight (f); f = new Font (fonts[i], Font.BOLD, 12); height += getHeight (f); f = new Font (fonts[i], Font.ITALIC, 12); height += getHeight (f); f = new Font (fonts[i], Font.BOLD | Font.ITALIC, 12); height += getHeight (f); maxWidth = Math.max (maxWidth, getWidth (f, fonts[i] + " BOLDITALIC")); } Insets inset = insets(); dim = new Dimension (maxWidth + inset.left + inset.right + hMargin, height + inset.top + inset.bottom + vMargin); resize (dim); } static public void main (String args[]) { Display f = new Display(); f.show(); } private int getHeight (Font f) { FontMetrics fm = Toolkit.getDefaultToolkit().getFontMetrics(f); return fm.getHeight(); } private int getWidth (Font f, String s) { FontMetrics fm = Toolkit.getDefaultToolkit().getFontMetrics(f); return fm.stringWidth(s); } public void paint (Graphics g) { int x = 0; int y = 0; g.translate(insets().left, insets().top); for (int i=0;i<fonts.length;i++) { Font plain = new Font (fonts[i], Font.PLAIN, 12); Font bold = new Font (fonts[i], Font.BOLD, 12); Font italic = new Font (fonts[i], Font.ITALIC, 12); Font bolditalic = new Font (fonts[i], Font.BOLD | Font.ITALIC, 12); g.setFont (plain); y += getHeight (plain); g.drawString (fonts[i] + " PLAIN", x, y); g.setFont (bold); y += getHeight (bold); g.drawString (fonts[i] + " BOLD", x, y); g.setFont (italic); y += getHeight (italic); g.drawString (fonts[i] + " ITALIC", x, y); g.setFont (bolditalic); y += getHeight (bolditalic); g.drawString (fonts[i] + " BOLDITALIC", x, y); } resize (dim); } }
Output:
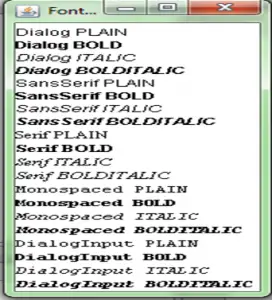